NEXT.js Part 1: Planning
Is it necessary to create another tutorial on using Next.js to create a web site? The goal of this introductory article is less about how to build a site using Next.js and more about the evaluation process of finding the right framework and platform based on your objectives.
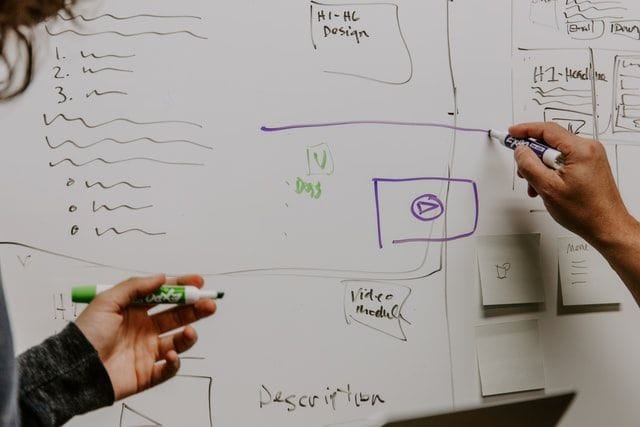
Welcome to the first of a multipart series on creating a web site using Next.js. If you want to jump straight to the development steps, that begins in Part Two.
This first post is intended for developers who may be less familiar with the technologies available for building a web site or (like me) find it difficult to select a framework when beginning a project. As the title indicates the conclusion for this series is choosing Next.js; that doesn't necessarily mean it is the right choice in every situation.
It is not an understatement to call deciding what technology stack to use to build a web site overwhelming. There are so many frameworks available today and any one of them will get the job done. In a way this actually does help make the process a little less complicated... as a developer you need to analyze what it is you are actually building. Start by answering two key questions:
What is the purpose of your site?
By purpose I mean more specifically the features and functionality that will be incorporated into the site. This is where you can identify if your site will be:
- Interactive: Users may have individual profiles, can work with data and/or save information. Think of online stores or possibly a social media site where users interact with each other. Applications like this require much more thought both on the overall experience as well as how to manage all of the parts of the application efficiently.
- Static: Users only interaction is reading content; possibly a showcase of your work or maybe just a blog for posting information.
Interactive and/or static is not necessarily an absolute decision, it is a sliding scale. Even if you are unsure and/or are planning to eventually build a large scale interactive web site it can be a great advantage to start small with the idea of adding complexity with feature additions over time. It is still important to look ahead, even at the beginning of your process; migrating an existing application between frameworks can be more difficult than building a new application in most instances.
Who will be responsible for updating the site?
If there are other users who will be maintaining content the next question is "What is the knowledge level of the users who will be maintaining this site?" If these users are not technical then there is likely going to be a need to provide tools for them to create and publish content in a simple way. On this site for example I am the only content contributor; building an interface to add/edit pages wouldn't be a priority.
I am going to touch on three options that could be viable depending on your evaluation of these questions. This is one opinion; there are no wrong answers when it comes to building a new web site; the key things you should focus on are your requirements and of course your level of comfort and experience as a developer.
WordPress is popular for very good reasons. WordPress has the ability to quickly stand up a functioning web site. It's also very end-user friendly; if you have non-technical users who will be managing some or all of the site content the tools provided by WordPress to do this are robust and easy to adopt. For developers there is significant power available behind the scenes; WordPress has a deep plugin architecture which allows you to buy or build functionality for your site. WordPress is often a hosted solution; this eliminates the need for standing up and maintaining the back end of a site on your own.
Angular is a framework created and maintained by Google - it is described as "the modern web developer's platform." Angular is extremely robust; at a high level is a module-based framework, meaning that you can build your application by creating individual modules that interact with each other and can even be reused across applications. Angular uses a View/Model approach; each component's functionality is managed in a code file and the presentation layer is managed in HTML file(s). Optionally the view can also incorporate CSS/SASS to control the styling of views. Angular is likely a good choice if you are building interactive applications with lots of user-friendly tools and features. It's also worth pointing out the Angular Material component library - this a very compelling argument for using Angular in advanced web applications - it is a vast library of ready-to-use components to quickly make your application look/feel professional. In my experience Angular is not as good a fit for developing simple sites. However if it is the tool set you are the most comfortable with then it's likely the best choice for your solution.
NEXT.js is a framework built on top of React; NEXT.js adds some of the features that are not incorporated into React including Typescript support (more on this later), routing and static site generation. Next.js is more comparable to Angular than React because of the features it adds. Chances are you visit a site powered by NEXT.js on a daily basis. You will find that overall Next.js is very similar in development experience to Angular, and the output is not going to be apparently different to your audience. There are countless articles, Reddit posts, and other information on the technical differences between Angular and Next.js. My experience with both would have me leaning in on NEXT.js for a simple web site:
- Next.js does support View/Model binding, but does not require physical page separation, so your model code and your template all live in the same page by default. I find this is easier and more intuitive to manage while still getting the model separation benefits.
- Next.js routing is much simpler to implement. The page you are viewing right now is a great example. The URL in your browser window ends in "site-building-pt-1" which was dynamically generated from the post title. In order to implement this in Next.js, you create a component with this syntax:
posts/[slug].tsx
. While there is more to wiring a route than just the name, fundamentally this is out of the box a working dynamic route in Next.js. - The overall experience of development in NEXT.js is comfortable to any developer who has a strong HTML/web development background. Most elements in your view are rendered using static HTML tags.
- The use of Angular Material is a compelling argument for many to lean towards Angular as a platform. React and Next.js by extension do have a comparable library - MUI. MUI is a component library that is also based on the Material UI design platform. MUI has a large set of components that can be leveraged for advanced features ranging from layouts to user interface elements and transitions.
Other Options
There are countless other frameworks available, all with their own advantages. Nothing should be off the table when considering the best solution to meet your specific needs. A few other options include:
- Vue.js Vue is similar to React in its approach of simplicity over feature, but also supports advanced features via additional libraries.
- Gatsby is another React-based framework. The Gatsby site notes a focus on performance and accessibility; overall the feature set is very similar to Next.js
- Spring.js is a Node/Express framework focused on rapid development of new applications.
- Django is another performance and simplicity-focused framework leveraging Python.